Let’s talk a bit about our first Creational Design Pattern, here is Singleton.
This pattern is common used when you want only one instance of an object.
Some frameworks such as Laravel have in your Service Container the possibility to register classes/objects as a singleton. It means that will have only one instance for these objects. However, let’s focus on their intent.
Critics consider the singleton to be an Anti-pattern in that it is frequently used in scenarios where it is not beneficial, introduces unnecessary restrictions in situations where a sole instance of a class is not actually required, and introduces global state into an application.
From Wikipedia
Don’t abuse using this pattern, use it only in cases where it should be really necessary.
It could be associated with Factory, Builder, and Prototype as well. It will have only one instance of factory that produces many products (Objects).
Intent
Ensures that a class has only one instance and provides a global access point for it.
Diagram
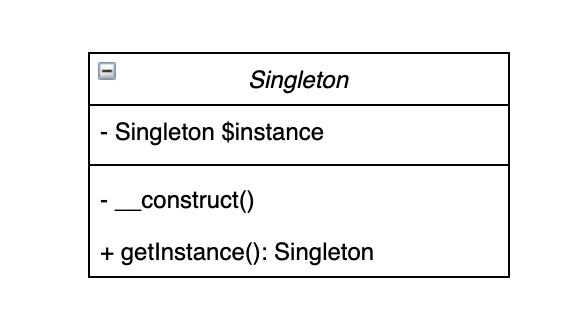
Our problem and solution
I though in a really simple example to represent when this could be used.
When I was working with a DebugBar, I had to collect information from different objects and to prevent that the information is missing or coming in wrong order I applied this pattern.
So for our example just apply this to a Logger class.
(Of course I won’t implement a full logger class here, because we’re focused only in the pattern, not in logging)
First let’s create right folders in our project, and it should be like this.
.designpatterns
.. src
... Creational
.... Logger
..... Logger.php
.. tests
... Creational
.... Logger
..... LoggerTest.php
Logger.php
<?php
declare(strict_types=1);
namespace DesignPatterns\Creational\Logger;
class Logger
{
private static $instance = null;
private function __construct() {}
public static function getInstance(): self
{
if (null === self::$instance) {
self::$instance = new self();
}
return self::$instance;
}
}
LoggerTest.php
<?php
declare(strict_types=1);
namespace Tests\Creational\Logger;
use DesignPatterns\Creational\Logger\Logger;
use PHPUnit\Framework\TestCase;
class LoggerTest extends TestCase
{
public function testItShouldBringTheSameInstance()
{
$logger = Logger::getInstance();
$logger2 = Logger::getInstance();
$this->assertEquals($logger, $logger2);
// If you do a var_dump() in these variables you will see that it's the same instance
}
}
You can follow our repository https://github.com/diegosm/designpatterns, everything is there.
I hope you understood this concept and feel free to ask something or do your contribution. There are many examples on internet of this pattern.
This article is a part of our Design Pattern Series, follow our Design Patterns โ What, when, why? link ๐
Leave a Reply